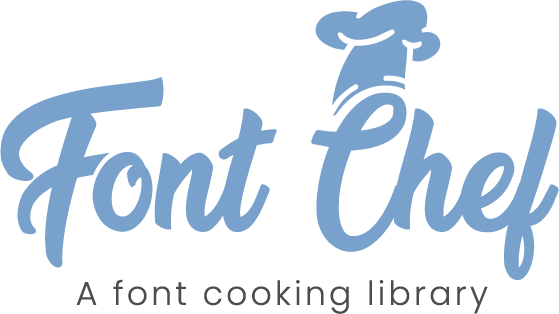 |
1.1.0
|
Complete example for the C API
- See on GitHub
- Checkout the common folder for the
state
implementation
#include "font-chef/font-chef.h"
#include "SDL.h"
#include "common/state.h"
#include "common/font.h"
int main(int argc, char const ** argv) {
struct state state = {};
init(&state);
struct texture * font_texture = texture_make(
&state,
);
const char text[] = "Hello, handsome! I mean... world!";
font,
(uint8_t *) text,
strlen(text),
state.bounds.right * 0.66f,
1.0f,
fc_align_left, mapping
);
while (update(&state)) {
render(font_texture, mapping[i].source, mapping[i].target);
}
}
texture_destroy(font_texture);
quit(&state);
return 0;
}
void fc_cook(struct fc_font *font)
Generates a bitmap and corresponding character information for a font.
struct fc_render_result fc_render_wrapped(struct fc_font const *font, unsigned char const *text, size_t byte_count, size_t line_width, float line_height_multiplier, enum fc_alignment alignment, struct fc_character_mapping *mapping)
A shortcut for rendering and then wrapping the result.
struct fc_font * fc_construct(uint8_t const *font_data, struct fc_font_size font_size, struct fc_color font_color)
Constructs a fc_font structure with the provided font, a size (either in pixels or points) and a font...
struct fc_font_size fc_pt(float value)
Constructs and returns a fc_size value specified as points.
A fc_font struct represents all data and metadata about a font.
void fc_destruct(struct fc_font *font)
Destroys and frees all memory allocated by this library.
void fc_add(struct fc_font *font, uint32_t first, uint32_t last)
Adds the given unicode range to the list of blocks to be cooked. You must add blocks before calling f...
A structure holding the result of a call to fc_render or fc_render_wrapped
Definition: font.h:49
struct fc_color const fc_color_white
RGBA: #FFFFFFFF
uint32_t last
The last unicode point in this range (inclusive)
Definition: unicode-block.h:26
uint32_t glyph_count
How many glphs were produced.
Definition: font.h:58
uint32_t first
The first unicode point in this range.
Definition: unicode-block.h:23
struct fc_pixels const * fc_get_pixels(struct fc_font const *font)
Returns the pixel data after for a font generated after a fc_cook was called.
Specifies source and target rectangles to render the specified codepoint.
Definition: character-mapping.h:34
void fc_move(struct fc_character_mapping *mapping, size_t count, float left, float baseline)
Moves all the target rectangles by left pixels horizontally and baseline pixels vertically.