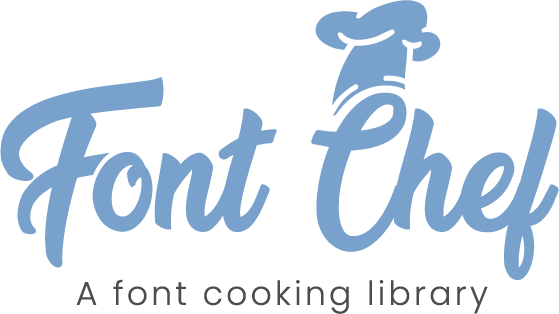 |
1.1.0
|
1 #ifndef FONT_CHEF_FONT_H
2 #define FONT_CHEF_FONT_H
15 #include "font-chef/font-chef-export.h"
16 #include "font-chef/unicode-block.h"
17 #include "font-chef/font-size.h"
18 #include "font-chef/character-mapping.h"
19 #include "font-chef/color.h"
20 #include "font-chef/size.h"
98 uint8_t
const * font_data,
132 FONT_CHEF_EXPORT
extern void fc_add(
214 unsigned char const * text,
242 unsigned char const * text,
245 float line_height_multiplier,
const uint8_t * fc_get_font_data(struct fc_font const *font)
Returns the font data specified in fc_construct.
struct fc_size dimensions
Width and height of this pixel data. Buffer size would be dimensions.width * dimensions....
Definition: font.h:43
fc_alignment
Specifies an aligment to be used when rendering wrapped text.
Definition: character-mapping.h:57
uint32_t line_count
How many lines were produced.
Definition: font.h:53
void fc_cook(struct fc_font *font)
Generates a bitmap and corresponding character information for a font.
struct fc_render_result fc_render_wrapped(struct fc_font const *font, unsigned char const *text, size_t byte_count, size_t line_width, float line_height_multiplier, enum fc_alignment alignment, struct fc_character_mapping *mapping)
A shortcut for rendering and then wrapping the result.
Specifies the size of a font either in pixels or in points.
Definition: font-size.h:33
Contains width and height as floats.
Definition: size.h:24
struct fc_font * fc_construct(uint8_t const *font_data, struct fc_font_size font_size, struct fc_color font_color)
Constructs a fc_font structure with the provided font, a size (either in pixels or points) and a font...
A fc_font struct represents all data and metadata about a font.
Provides information about a specific unicode block, as specified in ftp://ftp.unicode....
Definition: unicode-block.h:21
void fc_destruct(struct fc_font *font)
Destroys and frees all memory allocated by this library.
struct fc_color fc_get_color(struct fc_font const *font)
Returns the font color set to this font at construction time.
void fc_add(struct fc_font *font, uint32_t first, uint32_t last)
Adds the given unicode range to the list of blocks to be cooked. You must add blocks before calling f...
A structure holding the result of a call to fc_render or fc_render_wrapped
Definition: font.h:49
struct fc_unicode_block fc_get_block_at(struct fc_font const *font, size_t index)
Returns a unicode block at the index position.
struct fc_size fc_get_space_metrics(struct fc_font const *font)
Returns the space glyph width and height for this font at its specified size.
uint32_t glyph_count
How many glphs were produced.
Definition: font.h:58
size_t fc_get_block_count(struct fc_font const *font)
Returns count of added blocks in this font.
Represents a 4-byte-per-pixel color with red, green, blue and alpha components.
Definition: color.h:25
struct fc_pixels const * fc_get_pixels(struct fc_font const *font)
Returns the pixel data after for a font generated after a fc_cook was called.
struct fc_render_result fc_render(struct fc_font const *font, unsigned char const *text, size_t byte_count, struct fc_character_mapping *mapping)
Produces a list of source and target rectangles that can be used as clipping rects (or UV maps) for t...
struct fc_font_size fc_get_font_size(struct fc_font const *font)
Returns the font size set to this font at construction time.
Specifies source and target rectangles to render the specified codepoint.
Definition: character-mapping.h:34
A structure holding pixel data and its dimensions.
Definition: font.h:34
unsigned char * data
The pixel data produced after fc_cook, a 4-byte-per-pixel RGBA bitmap.
Definition: font.h:38