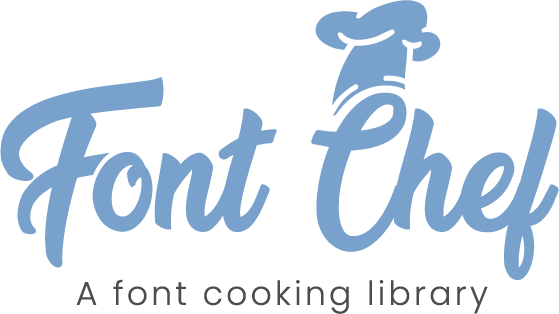 |
1.1.0
|
1 #ifndef FONT_CHEF_CHARACTER_MAPPING_H
2 #define FONT_CHEF_CHARACTER_MAPPING_H
4 #include "font-chef/size.h"
5 #include "font-chef/rect.h"
18 #include "font-chef/font-chef-export.h"
92 FONT_CHEF_EXPORT
extern uint32_t
fc_wrap(
fc_alignment
Specifies an aligment to be used when rendering wrapped text.
Definition: character-mapping.h:57
struct fc_rect fc_text_bounds(struct fc_character_mapping const mapping[], size_t length)
Calculates a bounding box for the rendered text.
struct fc_rect source
The source rectangle that should be used to clip the texture/surface.
Definition: character-mapping.h:38
struct fc_rect target
The target rectangle, or where in the target coordinates you should render the clipped texture.
Definition: character-mapping.h:42
float left
Left value.
Definition: rect.h:35
uint32_t fc_wrap(struct fc_character_mapping mapping[], size_t count, float line_width, float line_height, float space_width, enum fc_alignment alignment)
Word-wraps characters in an array of fc_character_mapping.
Represents a rectangle with left, top, right and bottom float values.
Definition: rect.h:33
uint32_t codepoint
Which unicode codepoint this mapping represents.
Definition: character-mapping.h:47
Specifies source and target rectangles to render the specified codepoint.
Definition: character-mapping.h:34
void fc_move(struct fc_character_mapping *mapping, size_t count, float left, float baseline)
Moves all the target rectangles by left pixels horizontally and baseline pixels vertically.