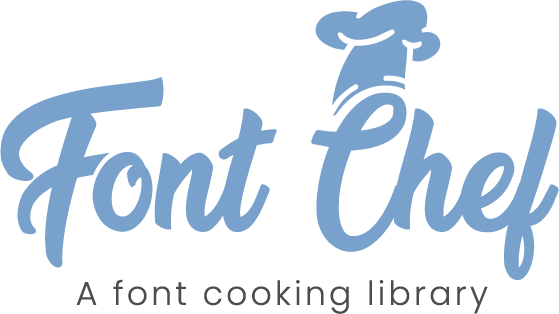 |
1.1.0
|
1 #ifndef FONT_CHEF_RENDER_RESULT_HPP
2 #define FONT_CHEF_RENDER_RESULT_HPP
4 #include "font-chef/character-mapping.h"
5 #include "font-chef/font.h"
33 std::vector<::fc_character_mapping>
mapping;
56 : mapping(mapping),
font(
font), line_count(0) {
64 : mapping(std::move(other.mapping)),
font(other.font), line_count(other.line_count) {
74 if (
this == &other)
return *
this;
75 this->mapping = std::move(other.mapping);
76 this->font = other.font;
77 this->line_count = other.line_count;
88 this->font = other.font;
89 if (
this == &other)
return *
this;
115 wrap(
float line_width,
float line_height_multiplier = 1.0f,
fc_alignment alignment = fc_align_left) & {
116 if (!
font)
return *
this;
123 space_metrics.
width * line_height_multiplier,
150 wrap(
float line_width,
float line_height_multiplier = 1.0f,
fc_alignment alignment = fc_align_left) && {
151 return std::move(wrap(line_width, line_height_multiplier, alignment));
170 fc_move(mapping.data(), mapping.size(), left, baseline);
189 return std::move(move(left, baseline));
196 std::vector<::fc_character_mapping>::iterator
begin() {
197 return mapping.begin();
204 std::vector<::fc_character_mapping>::iterator
end() {
205 return mapping.end();
213 return mapping.size();
Wraps a std::vector<fc_character_mapping>.
Definition: render-result.hpp:29
render_result & wrap(float line_width, float line_height_multiplier=1.0f, fc_alignment alignment=fc_align_left) &
Calls fc_wrap on the vector of character mappings.
Definition: render-result.hpp:115
fc_alignment
Specifies an aligment to be used when rendering wrapped text.
Definition: character-mapping.h:57
float height
The height value.
Definition: size.h:29
render_result && wrap(float line_width, float line_height_multiplier=1.0f, fc_alignment alignment=fc_align_left) &&
Calls fc_wrap on the vector of character mappings.
Definition: render-result.hpp:150
render_result & operator=(render_result const &other) noexcept
Copy assignment operator for fc::render_result.
Definition: render-result.hpp:87
Contains width and height as floats.
Definition: size.h:24
render_result(fc_font *font=nullptr, std::vector< fc_character_mapping > &&mapping={})
Constructs a fc::render_result instance with a font and with a mapping vector.
Definition: render-result.hpp:55
render_result & move(float left=0.0f, float baseline=0.0f) &
This is a wrapper to fc_move. Consult its documentation for more information.
Definition: render-result.hpp:169
size_t size() const
Returns the size of the mapping vector (i.e, the number of mappings)
Definition: render-result.hpp:212
A wrapper class for fc_font.
Definition: font.hpp:29
std::vector<::fc_character_mapping >::iterator begin()
Returns an iterator pointing to the first character mapping.
Definition: render-result.hpp:196
A fc_font struct represents all data and metadata about a font.
fc_font * font
A pointer to the fc_font used to generate this mapping.
Definition: render-result.hpp:43
font(font &&other) noexcept
Move constructor.
Definition: font.hpp:38
float width
The width value.
Definition: size.h:26
render_result & operator=(render_result &&other) noexcept
Move assignment operator for fc::render_result.
Definition: render-result.hpp:73
render_result(render_result &&other) noexcept
Move constructor of fc::render_result.
Definition: render-result.hpp:63
struct fc_size fc_get_space_metrics(struct fc_font const *font)
Returns the space glyph width and height for this font at its specified size.
uint32_t line_count
How many lines were produced.
Definition: render-result.hpp:38
uint32_t fc_wrap(struct fc_character_mapping mapping[], size_t count, float line_width, float line_height, float space_width, enum fc_alignment alignment)
Word-wraps characters in an array of fc_character_mapping.
render_result && move(float left=0.0f, float baseline=0.0f) &&
This is a wrapper to fc_move. Consult its documentation for more information.
Definition: render-result.hpp:188
std::vector<::fc_character_mapping >::iterator end()
Returns an iterator pointing to the end+1 character mapping.
Definition: render-result.hpp:204
void fc_move(struct fc_character_mapping *mapping, size_t count, float left, float baseline)
Moves all the target rectangles by left pixels horizontally and baseline pixels vertically.
std::vector<::fc_character_mapping > mapping
A vector of character mappings produced after calling fc::font::render.
Definition: render-result.hpp:33