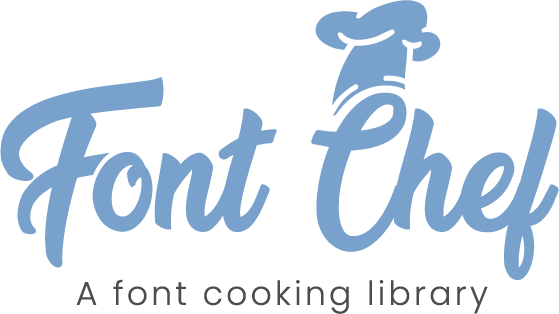 |
1.1.0
|
1 #ifndef FONT_CHEF_FONT_HPP
2 #define FONT_CHEF_FONT_HPP
9 #include "font-chef/font-chef-export.h"
10 #include "font-chef/font.h"
16 #include "font-chef/font-size.hpp"
17 #include "font-chef/color.hpp"
18 #include "font-chef/render-result.hpp"
29 class FONT_CHEF_EXPORT
font {
38 font(
font && other) noexcept : data(other.data) {
54 for (
size_t i = 0; i < block_count; i++) add(
fc_get_block_at(other.data, i));
86 return add(block.first, block.last);
100 return std::move(add(block.first, block.last));
114 fc_add(data, first, last);
129 font &&
add(uint32_t first, uint32_t last) && {
130 fc_add(data, first, last);
131 return std::move(*
this);
158 return std::move(*
this);
192 return std::move(render(text, result));
210 std::vector<fc_character_mapping> & mapping = result.
mapping;
211 if (mapping.size() < text.length()) mapping.resize(text.length());
244 #endif //FONT_CHEF_FONT_HPP
const uint8_t * fc_get_font_data(struct fc_font const *font)
Returns the font data specified in fc_construct.
Wraps a std::vector<fc_character_mapping>.
Definition: render-result.hpp:29
uint32_t line_count
How many lines were produced.
Definition: font.h:53
void fc_cook(struct fc_font *font)
Generates a bitmap and corresponding character information for a font.
struct fc_font * fc_construct(uint8_t const *font_data, struct fc_font_size font_size, struct fc_color font_color)
Constructs a fc_font structure with the provided font, a size (either in pixels or points) and a font...
A wrapper class for fc_color.
Definition: color.hpp:18
font && add(fc_unicode_block const &block) &&
Adds a new unicode block to be cooked.
Definition: font.hpp:99
A wrapper class for fc_font.
Definition: font.hpp:29
font & add(uint32_t first, uint32_t last) &
Adds a new unicode range to be cooked.
Definition: font.hpp:113
A fc_font struct represents all data and metadata about a font.
Provides information about a specific unicode block, as specified in ftp://ftp.unicode....
Definition: unicode-block.h:21
void fc_destruct(struct fc_font *font)
Destroys and frees all memory allocated by this library.
struct fc_color fc_get_color(struct fc_font const *font)
Returns the font color set to this font at construction time.
void fc_add(struct fc_font *font, uint32_t first, uint32_t last)
Adds the given unicode range to the list of blocks to be cooked. You must add blocks before calling f...
A structure holding the result of a call to fc_render or fc_render_wrapped
Definition: font.h:49
fc_pixels pixels() const
Obtains a structure containing a pointer to the pixel data and it's dimensions.
Definition: font.hpp:169
fc_font * font
A pointer to the fc_font used to generate this mapping.
Definition: render-result.hpp:43
font(font &&other) noexcept
Move constructor.
Definition: font.hpp:38
fc::render_result & render(std::string const &text, fc::render_result &result) const
Produces clipping and target rectangles to render specified text reusing an instance of fc::render_re...
Definition: font.hpp:208
font & cook() &
Cooks all the added unicode ranges into a pixmap and clipping information.
Definition: font.hpp:142
struct fc_unicode_block fc_get_block_at(struct fc_font const *font, size_t index)
Returns a unicode block at the index position.
font && add(uint32_t first, uint32_t last) &&
Adds a new unicode range to be cooked.
Definition: font.hpp:129
font from(uint8_t const *font_data, fc::font_size const &font_size, fc::color const &font_color)
A helper method to ease font cooking via method chaining.
Definition: font.hpp:238
font && cook() &&
Cooks all the added unicode ranges into a pixmap and clipping information.
Definition: font.hpp:156
fc::render_result render(std::string const &text) const
Produces clipping and target rectangles to render specified text.
Definition: font.hpp:190
uint32_t line_count
How many lines were produced.
Definition: render-result.hpp:38
uint32_t glyph_count
How many glphs were produced.
Definition: font.h:58
size_t fc_get_block_count(struct fc_font const *font)
Returns count of added blocks in this font.
~font()
Destructs a fc::font instance and frees all the memory associated with it.
Definition: font.hpp:72
struct fc_pixels const * fc_get_pixels(struct fc_font const *font)
Returns the pixel data after for a font generated after a fc_cook was called.
struct fc_render_result fc_render(struct fc_font const *font, unsigned char const *text, size_t byte_count, struct fc_character_mapping *mapping)
Produces a list of source and target rectangles that can be used as clipping rects (or UV maps) for t...
struct fc_font_size fc_get_font_size(struct fc_font const *font)
Returns the font size set to this font at construction time.
A wrapper class for fc_font_size.
Definition: font-size.hpp:18
font & add(fc_unicode_block const &block) &
Adds a new unicode block to be cooked.
Definition: font.hpp:85
font(font const &other)
Copy constructor.
Definition: font.hpp:47
A structure holding pixel data and its dimensions.
Definition: font.h:34
font(uint8_t const *font_data, fc::font_size const &font_size, fc::color const &font_color)
Constructs a fc::font instance from a font data, a font size and a font color.
Definition: font.hpp:65
std::vector<::fc_character_mapping > mapping
A vector of character mappings produced after calling fc::font::render.
Definition: render-result.hpp:33