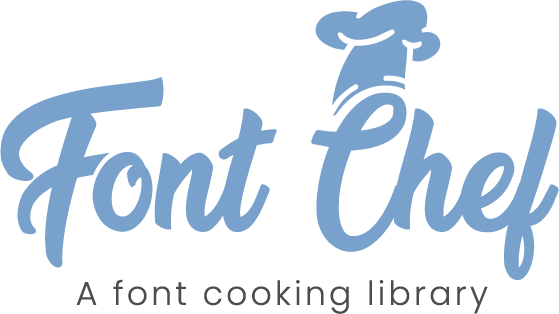 |
1.1.0
|
1 #ifndef FONT_CHEF_FONT_SIZE_HPP
2 #define FONT_CHEF_FONT_SIZE_HPP
9 #include "font-chef/font-chef-export.h"
10 #include "font-chef/font-size.h"
75 #endif //FONT_CHEF_FONT_SIZE_HPP
font_size(fc_font_size const &fs)
Creates a fc::font_size instance from a fc_font_size structure, copying its contents.
Definition: font-size.hpp:36
Specifies the size of a font either in pixels or in points.
Definition: font-size.h:33
font_size(float value, enum fc_font_size_type type)
Constructs a font size from a value and a type.
Definition: font-size.hpp:49
fc_font_size_type
Used to tell if a fc_font_size value is specified in pixels or in points.
Definition: font-size.h:24
font_size px(float v)
Constructs a fc::font_size instance in pixels with specified value.
Definition: font-size.hpp:59
font_size()
Definition: font-size.hpp:25
font_size pt(float v)
Constructs a fc::font_size instance in points with specified value.
Definition: font-size.hpp:70
fc_font_size data
the actual fc_font_size data. You can and are encouraged to use it directly, if needed.
Definition: font-size.hpp:22
A wrapper class for fc_font_size.
Definition: font-size.hpp:18