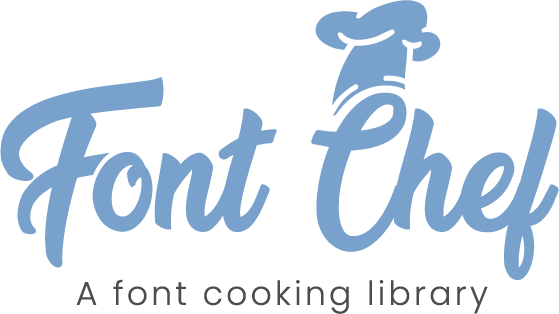 |
1.1.0
|
1 #ifndef FONT_CHEF_COLOR_HPP
2 #define FONT_CHEF_COLOR_HPP
9 #include "font-chef/font-chef-export.h"
10 #include "font-chef/color.h"
49 color(uint8_t r, uint8_t g, uint8_t b, uint8_t a = 255) : data{r, g, b, a} { }
55 uint8_t
r()
const {
return data.
r; }
61 uint8_t
g()
const {
return data.
g; }
67 uint8_t
b()
const {
return data.
b; }
73 uint8_t
a()
const {
return data.
a; }
79 color &
r(uint8_t v) { data.
r = v;
return *
this; }
85 color &
g(uint8_t v) { data.
g = v;
return *
this; }
91 color &
b(uint8_t v) { data.
b = v;
return *
this; }
97 color &
a(uint8_t v) { data.
a = v;
return *
this; }
122 FONT_CHEF_EXPORT
inline color rgba(uint8_t r, uint8_t g, uint8_t b, uint8_t a) {
123 return color(r, g, b, a);
135 FONT_CHEF_EXPORT
inline color rgb(uint8_t r, uint8_t g, uint8_t b) {
136 return color(r, g, b);
152 #endif //FONT_CHEF_COLOR_HPP
uint8_t r
Definition: color.h:27
color rgba(uint8_t r, uint8_t g, uint8_t b, uint8_t a)
Constructs a fc::color instance from red, green, blue and alpha values.
Definition: color.hpp:122
color & a(uint8_t v)
Sets the alpha component for this color.
Definition: color.hpp:97
A wrapper class for fc_color.
Definition: color.hpp:18
color rgb(uint32_t c)
Constructs a fc::color instance from an integer with red, green and blue components,...
Definition: color.hpp:146
color & b(uint8_t v)
Sets the blue component for this color.
Definition: color.hpp:91
color(fc_color const &c)
Creates a fc::color instance from a fc_color value.
Definition: color.hpp:33
color & g(uint8_t v)
Sets the green component for this color.
Definition: color.hpp:85
uint8_t g() const
Gets the green component for this color.
Definition: color.hpp:61
uint8_t b() const
Gets the blue component for this color.
Definition: color.hpp:67
color & r(uint8_t v)
Sets the red component for this color.
Definition: color.hpp:79
uint8_t g
Definition: color.h:30
uint8_t r() const
Gets the red component for this color.
Definition: color.hpp:55
uint8_t a
Definition: color.h:36
uint8_t a() const
Gets the alpha component for this color.
Definition: color.hpp:73
Represents a 4-byte-per-pixel color with red, green, blue and alpha components.
Definition: color.h:25
color(uint8_t r, uint8_t g, uint8_t b, uint8_t a=255)
Constructs a fc::color instance from red, green, blue and alpha components.
Definition: color.hpp:49
color()
Definition: color.hpp:25
fc_color data
The internal fc_color value.
Definition: color.hpp:22
struct fc_color fc_0xrgba(uint32_t color)
Constructs and return a fc_color value with red, green and blue components extracted from an unsigned...
uint8_t b
Definition: color.h:33
struct fc_color fc_0xrgb(uint32_t color)
Constructs and return a fc_color value with red, green and blue components extracted from an unsigned...