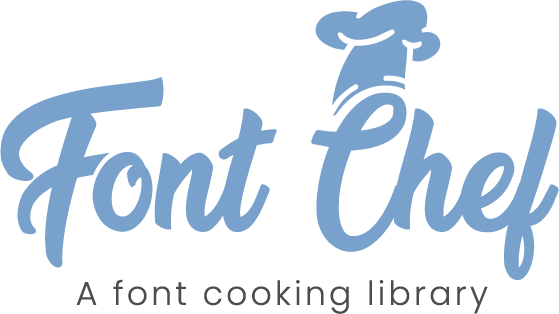 |
1.1.0
|
1 #ifndef FONT_CHEF_COLOR_H
2 #define FONT_CHEF_COLOR_H
14 #include "font-chef/font-chef-export.h"
71 FONT_CHEF_EXPORT
extern void fc_color_set(
struct fc_color * color, uint8_t r, uint8_t g, uint8_t b, uint8_t a);
88 FONT_CHEF_EXPORT
extern struct fc_color fc_rgba(uint8_t
r, uint8_t
g, uint8_t
b, uint8_t
a);
uint8_t r
Definition: color.h:27
struct fc_color const fc_color_black
RGBA: #000000FF
struct fc_color fc_rgba(uint8_t r, uint8_t g, uint8_t b, uint8_t a)
Constructs and return a fc_color value with the provided components set.
struct fc_color const fc_color_red
RGBA: #FF0000FF
struct fc_color const fc_color_white
RGBA: #FFFFFFFF
uint8_t g
Definition: color.h:30
struct fc_color fc_rgb(uint8_t r, uint8_t g, uint8_t b)
Constructs and return a fc_color value with the provided components set and alpha set as 255 (opaque)
uint8_t a
Definition: color.h:36
Represents a 4-byte-per-pixel color with red, green, blue and alpha components.
Definition: color.h:25
struct fc_color const fc_color_blue
RGBA: #0000FFFF
struct fc_color fc_0xrgba(uint32_t color)
Constructs and return a fc_color value with red, green and blue components extracted from an unsigned...
void fc_color_set(struct fc_color *color, uint8_t r, uint8_t g, uint8_t b, uint8_t a)
Sets all fields of a fc_color structure at once.
struct fc_color const fc_color_green
RGBA: #00FF00FF
uint8_t b
Definition: color.h:33
struct fc_color fc_0xrgb(uint32_t color)
Constructs and return a fc_color value with red, green and blue components extracted from an unsigned...
void fc_color_construct(struct fc_color *color)
Zeroes all fields of a fc_color structure.